【動作環境】
<パソコン>
機種:MacBook Air(13inch)
OS:macOS Sonoma(ver.14.5)
チップ:Apple M3
シェル:zsh
<ソフトウェア>
・Flutter(ver.3.22.3)
・Xcode(ver.15.4)
・CocoaPods(ver.1.15.2)
・Android Studio(ver.2024.1)
・Visual Studio Code(ver.1.92.0)
今回は、Flutterでテキストを表示する方法について解説します。
準備
まずは、main.dart
に以下のコードを書き込みましょう。
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(title),
),
body: const Text('You have pushed the button this many times:'),
);
}
}
iOSシミュレーターで実行すると、以下のようになります。
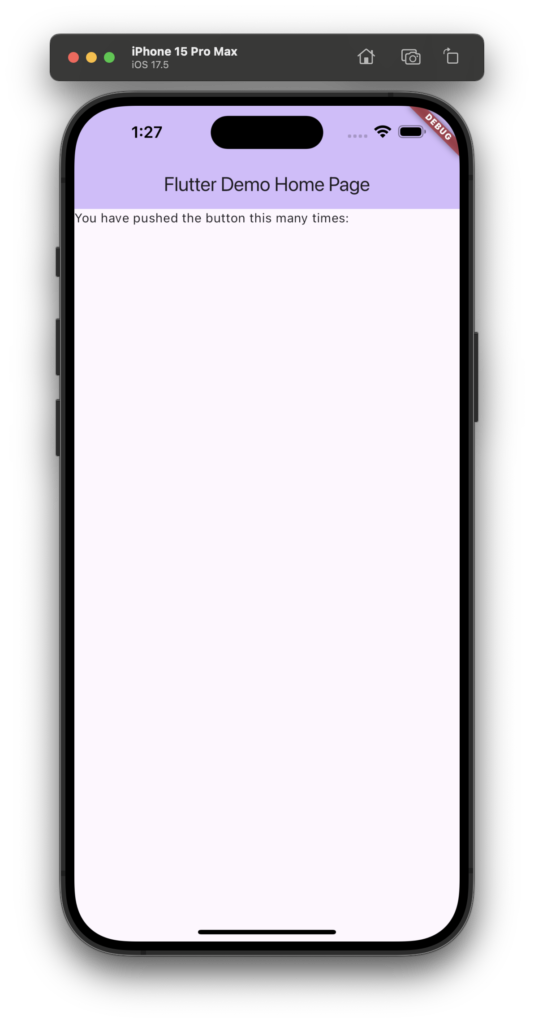
テキストを表示する
Flutterでは、Textウィジェットを使用することで、簡単にテキストを表示することができます。
AppBarのタイトルを変更する
AppBarウィジェットのtitleプロパティにTextウィジェットを設置することで、AppBarのタイトルを表示することができます。
すでに、Textウィジェットが設置されているので、ウィジェットの中身を変えてみましょう。
title: Text(title),
title: const Text('知恵袋ゼロ'),
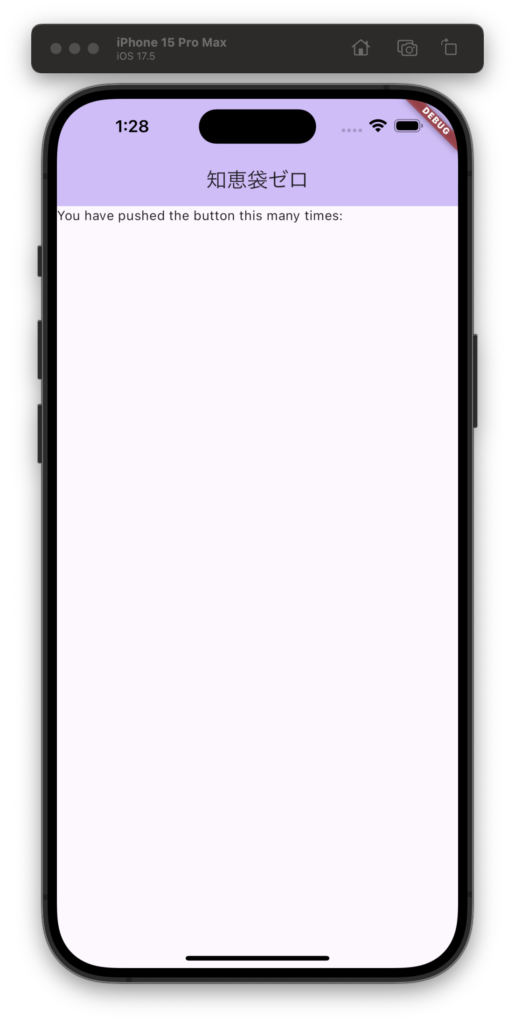
文字列を入力する際は、「’(クォーテーション)」で囲むようにしましょう。
Bodyのテキストを変更する
次に、Scaffoldウィジェットのbodyプロパティに設置したTextウィジェットの中身を変えてみましょう。
body: const Text('You have pushed the button this many times:'),
body: const Text('テキストを表示する時は、Textウィジェットを使います'),
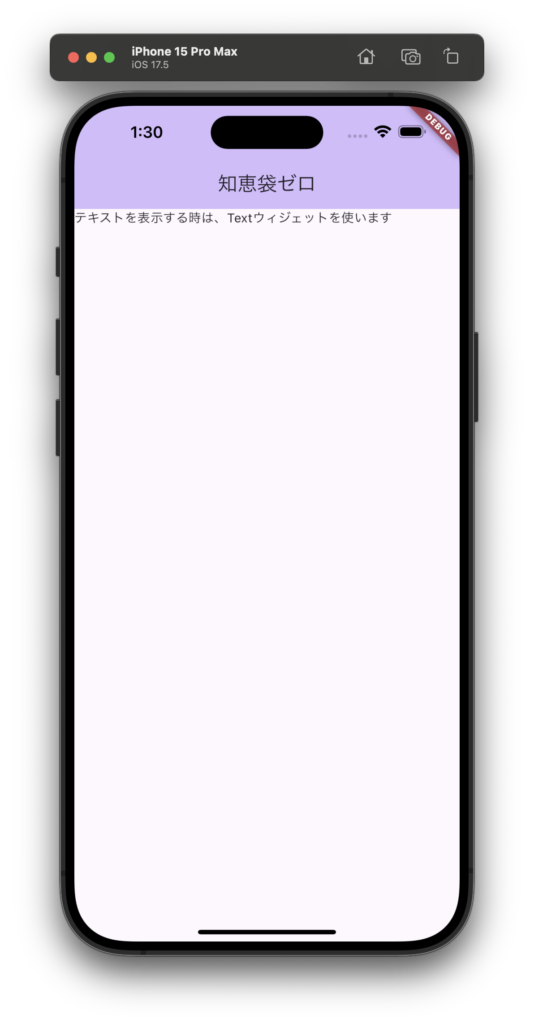
テキストの値は、基本的に変化しないため、「const」という定数を示す修飾子をTextウィジェットの前につけます。
そうすることで、アプリの動作が若干早くなります。
つけない場合は注意メッセージが表示されますが、動作上に問題はありません。
テキストのスタイルを変更する
Textウィジェットでは、styleプロパティにTextStyleウィジェットを設置することで、文字の大きさや色、太さなどを変更することができます。
文字の大きさを変更する
TextStyleウィジェットにfontSizeプロパティ設置して、値を入力します。
body: const Text('テキストを表示する時は、Textウィジェットを使います'),
);
}
}
body: const Text(
'テキストを表示する時は、Textウィジェットを使います',
style: TextStyle(fontSize: 24),
),
);
}
}
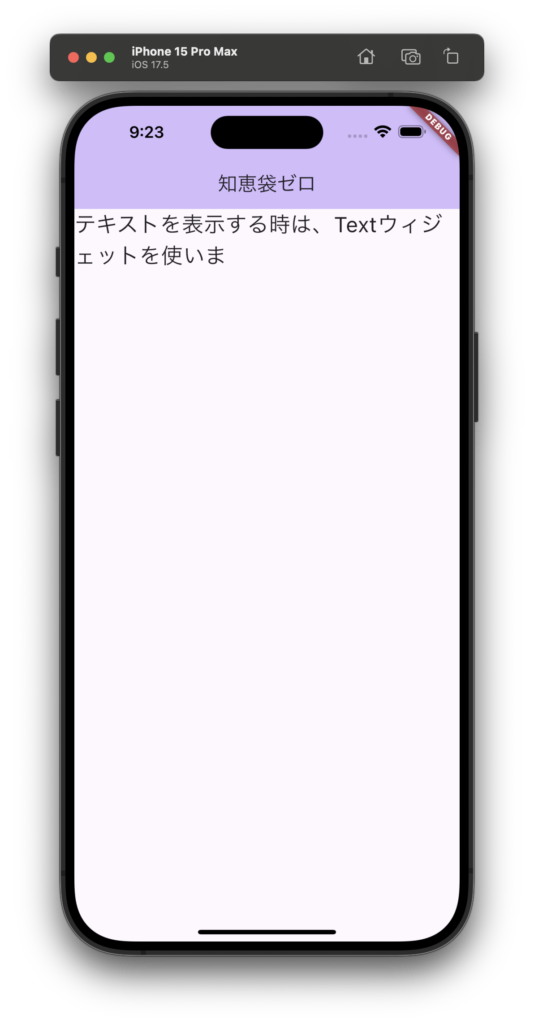
文字色を変更する
TextStyleウィジェットにcolorプロパティ設置します。
そして、Colorsウィジェットにblueプロパティを設置します。
body: const Text(
'テキストを表示する時は、Textウィジェットを使います',
style: TextStyle(fontSize: 24),
),
);
}
}
body: const Text(
'テキストを表示する時は、Textウィジェットを使います',
style: TextStyle(
fontSize: 24,
color: Colors.blue,
),
),
);
}
}
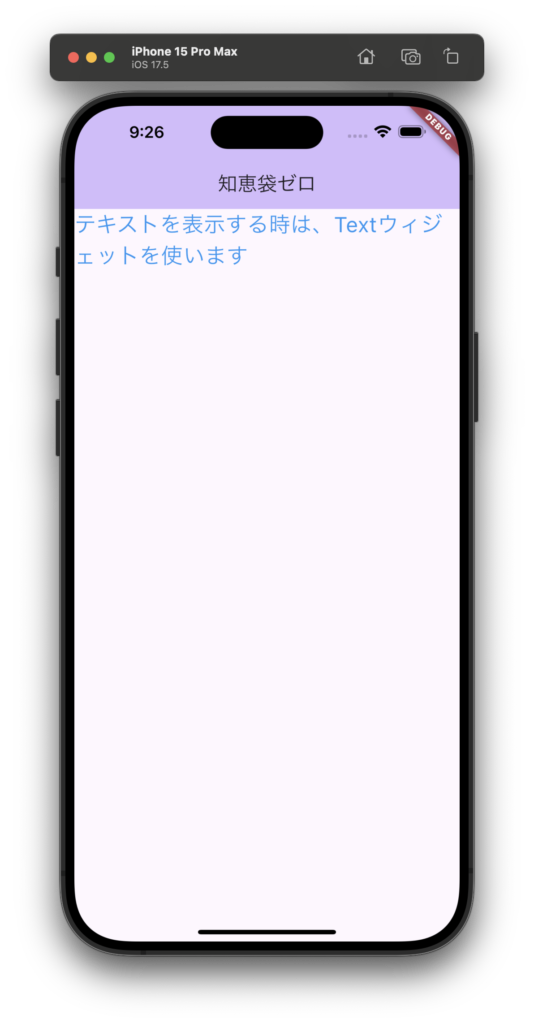
文字の太さを変更する
TextStyleウィジェットにfontWeightプロパティ設置します。
そして、FontWeightウィジェットにboldプロパティを設置します。
body: const Text(
'テキストを表示する時は、Textウィジェットを使います',
style: TextStyle(
fontSize: 24,
color: Colors.blue,
),
),
);
}
}
body: const Text(
'テキストを表示する時は、Textウィジェットを使います',
style: TextStyle(
fontSize: 24,
color: Colors.blue,
fontWeight: FontWeight.bold,
),
),
);
}
}
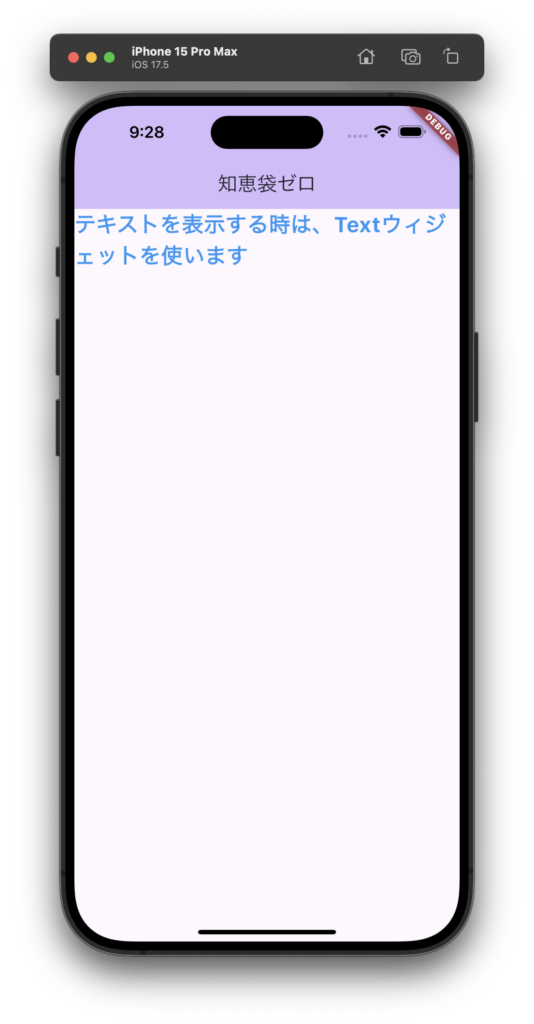
文字をイタリックにする
TextStyleウィジェットにfontStyleプロパティ設置します。
そして、FontStyleウィジェットにitalicプロパティを設置します。
body: const Text(
'テキストを表示する時は、Textウィジェットを使います',
style: TextStyle(
fontSize: 24,
color: Colors.blue,
fontWeight: FontWeight.bold,
),
),
);
}
}
body: const Text(
'テキストを表示する時は、Textウィジェットを使います',
style: TextStyle(
fontSize: 24,
color: Colors.blue,
fontWeight: FontWeight.bold,
fontStyle: FontStyle.italic,
),
),
);
}
}
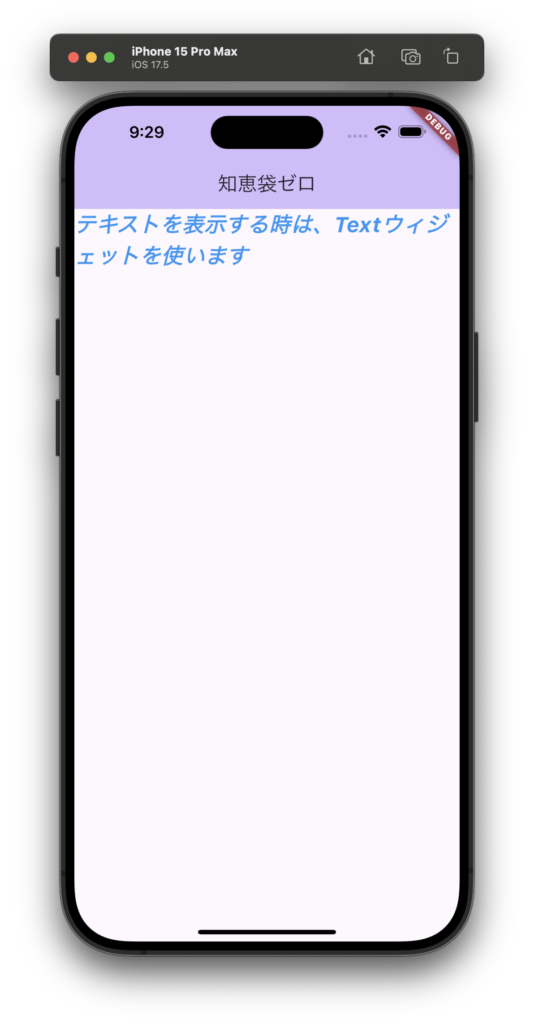
文字にアンダーラインを追加する
TextStyleウィジェットにdecorationプロパティ設置します。
そして、TextDecorationウィジェットにunderlineプロパティを設置します。
body: const Text(
'テキストを表示する時は、Textウィジェットを使います',
style: TextStyle(
fontSize: 24,
color: Colors.blue,
fontWeight: FontWeight.bold,
fontStyle: FontStyle.italic,
),
),
);
}
}
body: const Text(
'テキストを表示する時は、Textウィジェットを使います',
style: TextStyle(
fontSize: 24,
color: Colors.blue,
fontWeight: FontWeight.bold,
fontStyle: FontStyle.italic,
decoration: TextDecoration.underline,
),
),
);
}
}
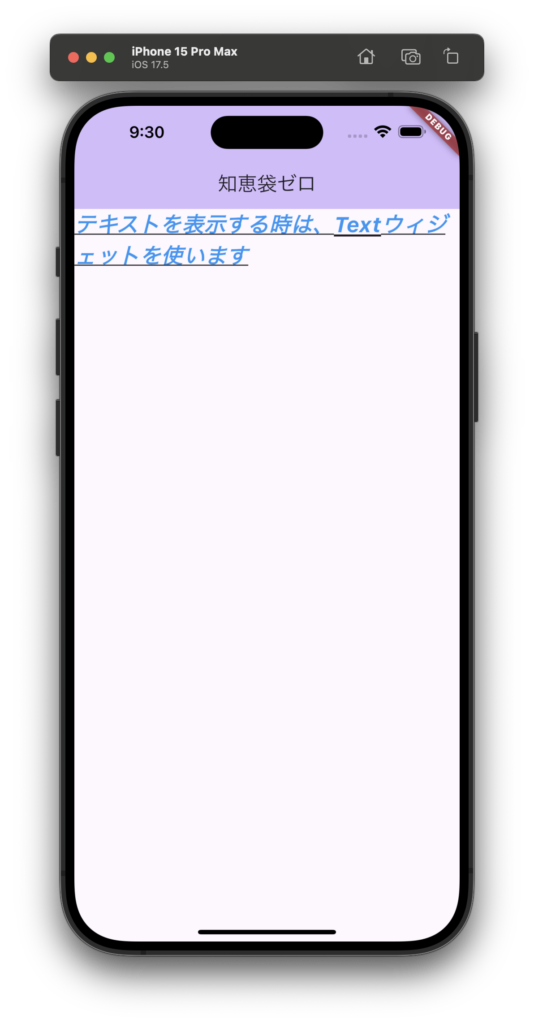
コンテナ内におけるテキストの位置を変更する
先ほどは、Textウィジェットにstyleプロパティを設置して、そこにTextStyleウィジェットを設置して、テキストの装飾を行いました。
次は、TextウィジェットにtextAlignプロパティを設置して、そこにTextAlignウィジェット設置して、テキストの配置を変更していきます。
ただ、TextAlignウィジェットを設置するだけでは、変化がわかりにくいので、TextウィジェットをContainerウィジェットで包みます。
まずは、以下のように35行目以下の部分を書き換えます。
body: const Text(
'テキストを表示する時は、Textウィジェットを使います',
),
);
}
}
そして、TextウィジェットをContainerウィジェットで包み、見た目がわかりやすくなるようにContainerウィジェットの大きさと色を変更します。
body: Container(
width: double.infinity,
color: Colors.grey,
child: const Text(
'テキストを表示する時は、Textウィジェットを使います',
),
),
);
}
}
簡単にウィジェットを別のウィジェットで包む方法
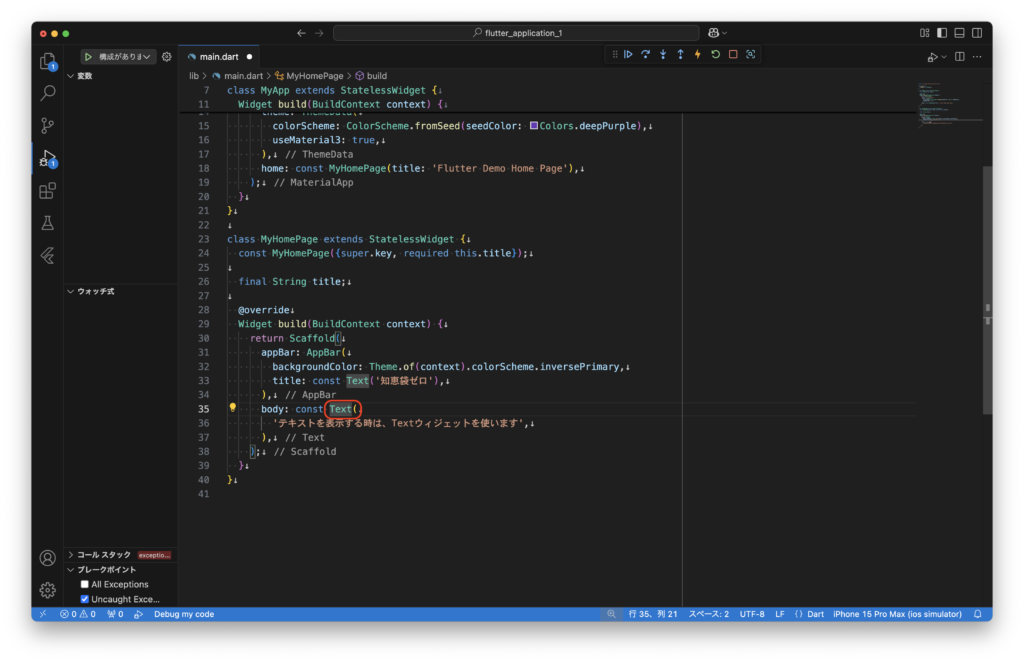
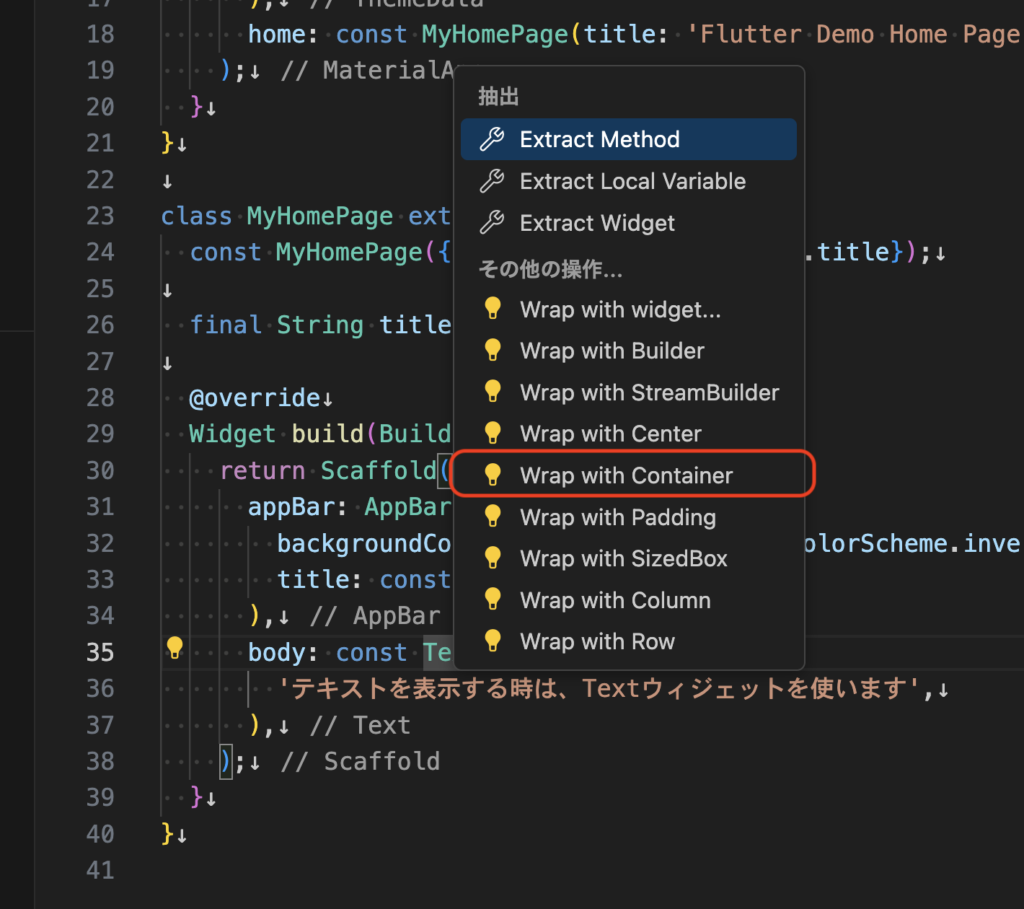
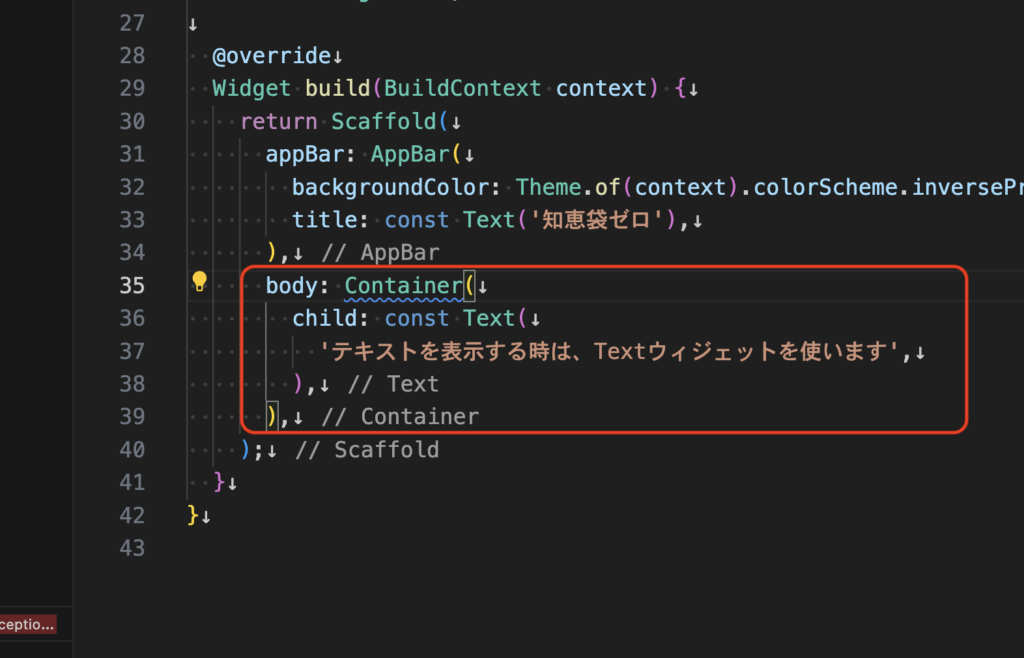
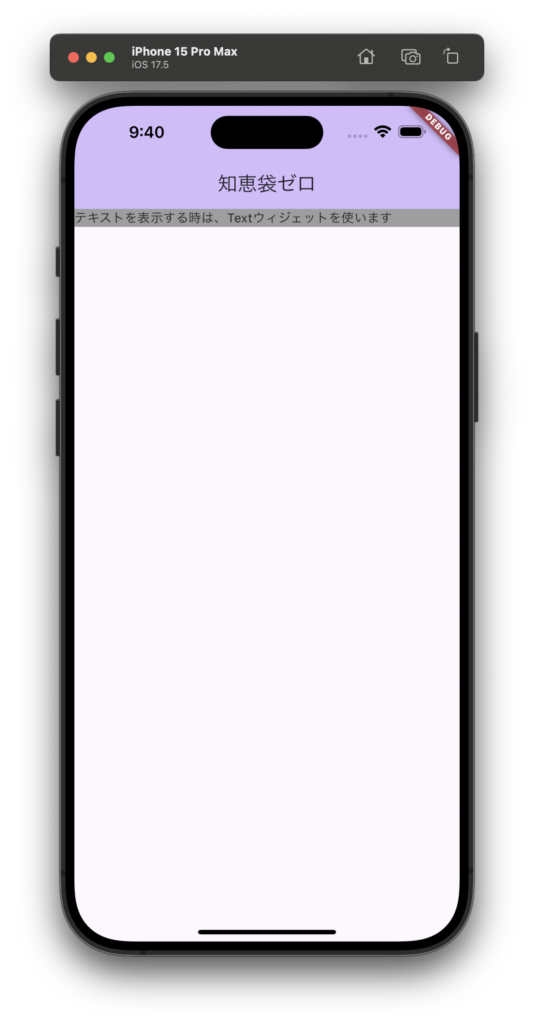
右寄せにする
TextウィジェットにtextAlignプロパティ設置します。
そして、TextAlignウィジェットを設置し、rightプロパティを設置します。
body: Container(
width: double.infinity,
color: Colors.grey,
child: const Text(
'テキストを表示する時は、Textウィジェットを使います',
),
),
);
}
}
body: Container(
width: double.infinity,
color: Colors.grey,
child: const Text(
'テキストを表示する時は、Textウィジェットを使います',
textAlign: TextAlign.right,
),
),
);
}
}
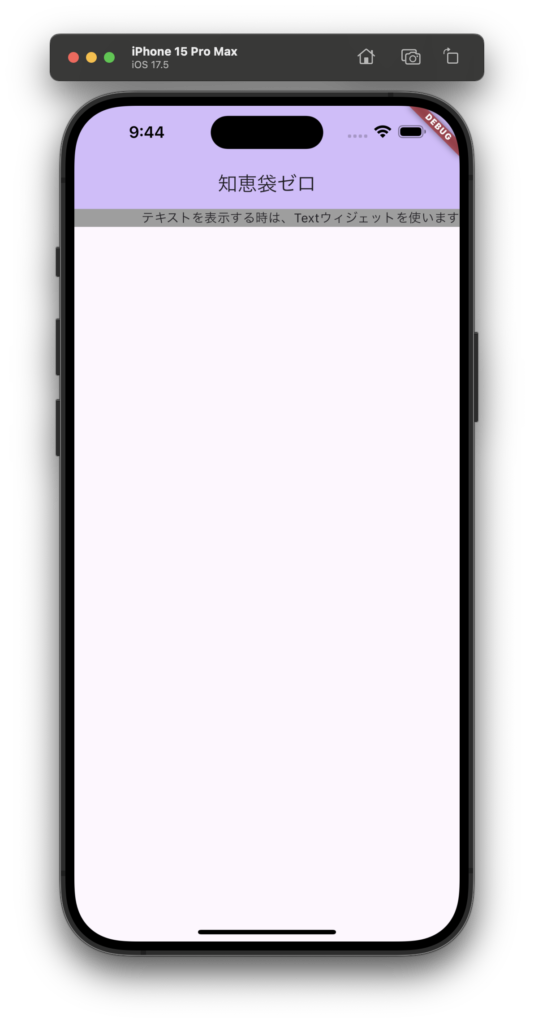
中央寄せにする
TextAlignウィジェットにcenterプロパティを設置します。
body: Container(
width: double.infinity,
color: Colors.grey,
child: const Text(
'テキストを表示する時は、Textウィジェットを使います',
textAlign: TextAlign.right,
),
),
);
}
}
body: Container(
width: double.infinity,
color: Colors.grey,
child: const Text(
'テキストを表示する時は、Textウィジェットを使います',
textAlign: TextAlign.center,
),
),
);
}
}
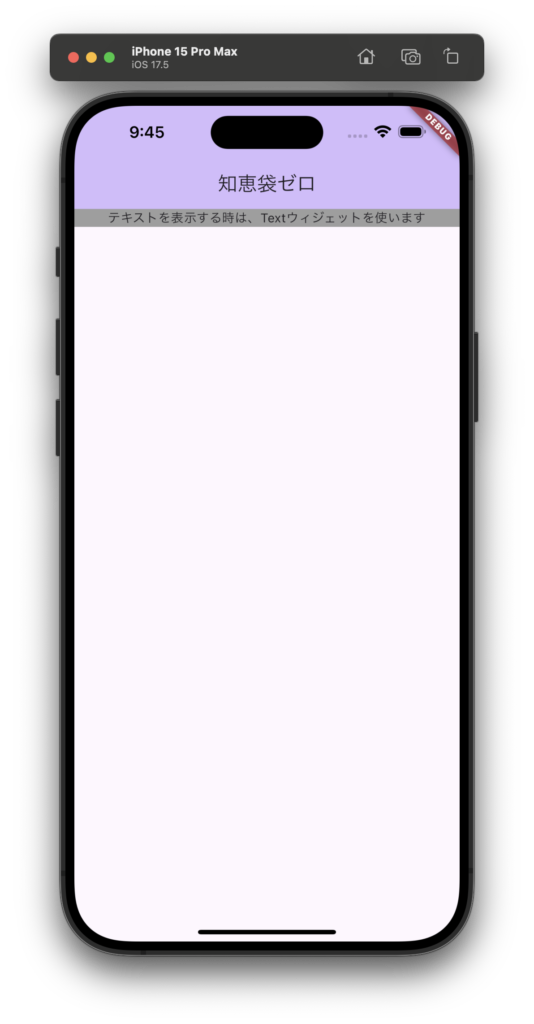
左寄せにする
TextAlignウィジェットにleftプロパティを設置します。
body: Container(
width: double.infinity,
color: Colors.grey,
child: const Text(
'テキストを表示する時は、Textウィジェットを使います',
textAlign: TextAlign.center,
),
),
);
}
}
body: Container(
width: double.infinity,
color: Colors.grey,
child: const Text(
'テキストを表示する時は、Textウィジェットを使います',
textAlign: TextAlign.left,
),
),
);
}
}
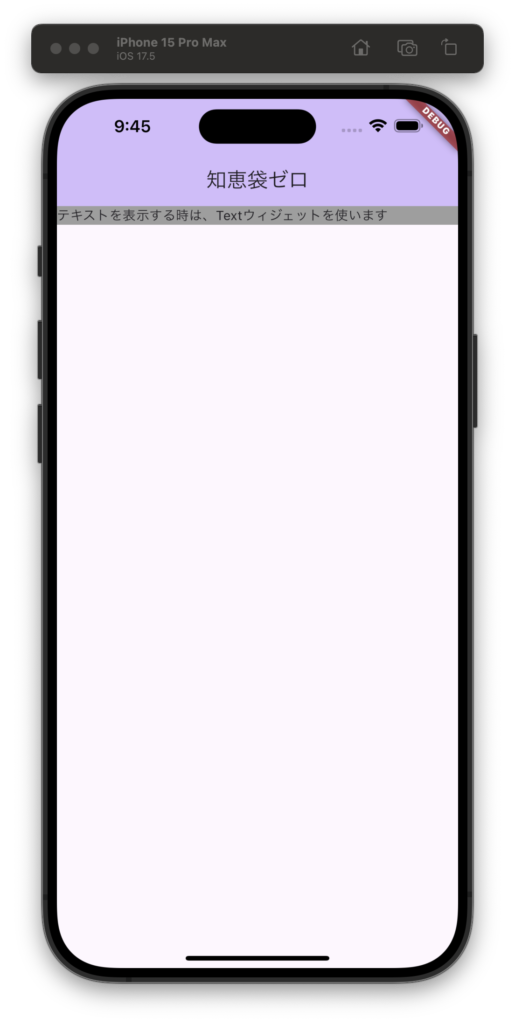
まとめ
この記事では、Flutterを使ったテキスト表示の基本について解説しました。以下のポイントを取り上げました。
- AppBarとBodyのテキスト表示:基本的なテキストの表示方法と変更方法を学びました。
- テキストスタイルの変更:文字サイズや色、太さ、装飾をカスタマイズする方法を学びました。
- コンテナ内でのテキスト配置:ContainerウィジェットとTextAlignウィジェットを組み合わせて、テキストの配置を変更する方法を学びました。
これらの知識を活用して、アプリのテキスト表示をより魅力的にデザインしてみてください。
ウィジェットをさらに使いこなすことで、様々な表現が可能になります。
コメント